JavaScript console.log() All Details With Example.
Hello everyone,
Today I'm going to talk about one of the most useful tools for debugging JavaScript code: console.log().
console.log() is a way to print out any data you have in your program, so that you can check it and make sure everything is working as expected.
So how does console.log() work?
Well, let’s say we have some simple JavaScript code like the following example.
const text = "Hello World"; console.log(text)
- Declare a variable called 'text' with value 'Hello World'.
- Print out the value of 'text' using the console.log() function.
In this example, when we run our script the output would be 'Hello World' printed on our screen (browser console).
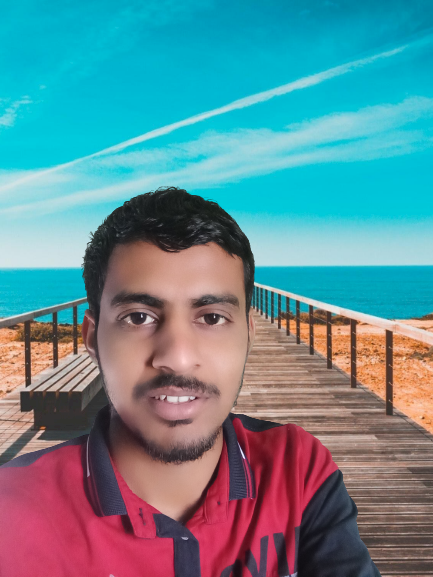

This happens because when we use console.log() function, it prints whatever argument or data that comes after it. In this case, its printing out the value stored inside the 'text' variable which was declared earlier as 'Hello World' .
So if something isn't working correctly in our program then first thing should be checking what values are being passed around by using console.log() function.We can also pass multiple arguments into the console.log() function by separating them with commas.
const text = "Hello World"; const number = 55; console.log(text, number)
const myObj = {firstname:"Apu", age:"24"};
console.log(myObj);
Write an array to the console.
const myArr = ["Red", "Green", "Blue", "White"];
console.log(myArr);
const myObj = {
firstname:"Apu",
age:"24"
};
console.log(myObj);
Write an array to the console.
const myArr = [
"Red",
"Green",
"Blue",
"White"
];
console.log(myArr);
const myObj = {
firstname:"Apu",
age:"24"
};
console.log(myObj);
Write an array to the console.
const myArr = [
"Red",
"Green",
"Blue",
"White"
];
console.log(myArr);
const text = "Hello World";
const number = 55;
console.log(text, number)
const text = "Hello World";
const number = 55;
console.log(text, number)